Embedded Integration Guide
The following guide will walk you through step-by-step how to integrate and test the Memfault SDK for a Cortex-M device using the GNU GCC, Clang, IAR, ARM MDK, or TI ARM Compiler.
Adding the Memfault Firmware SDK to your device will provide rich diagnostics, including:
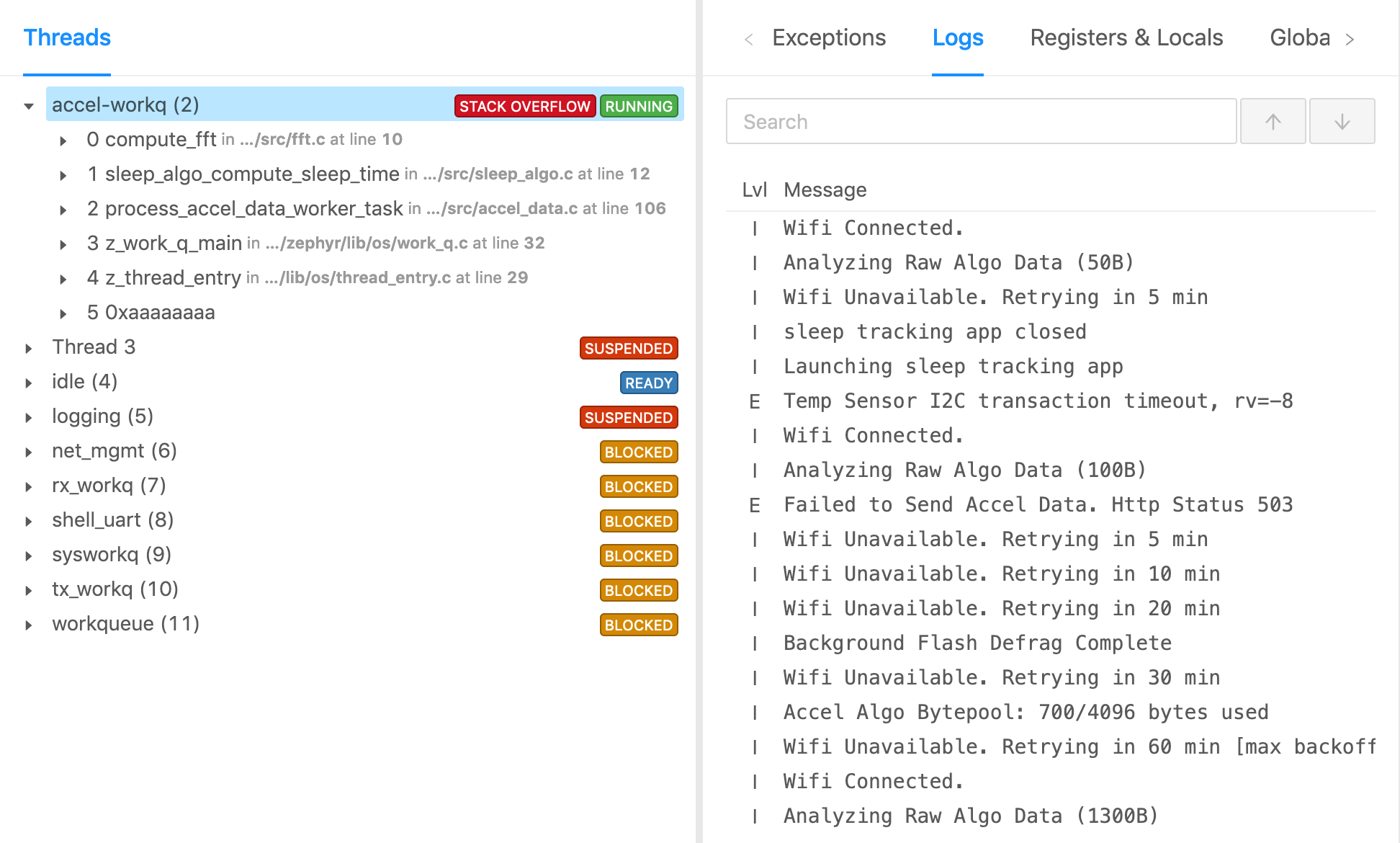
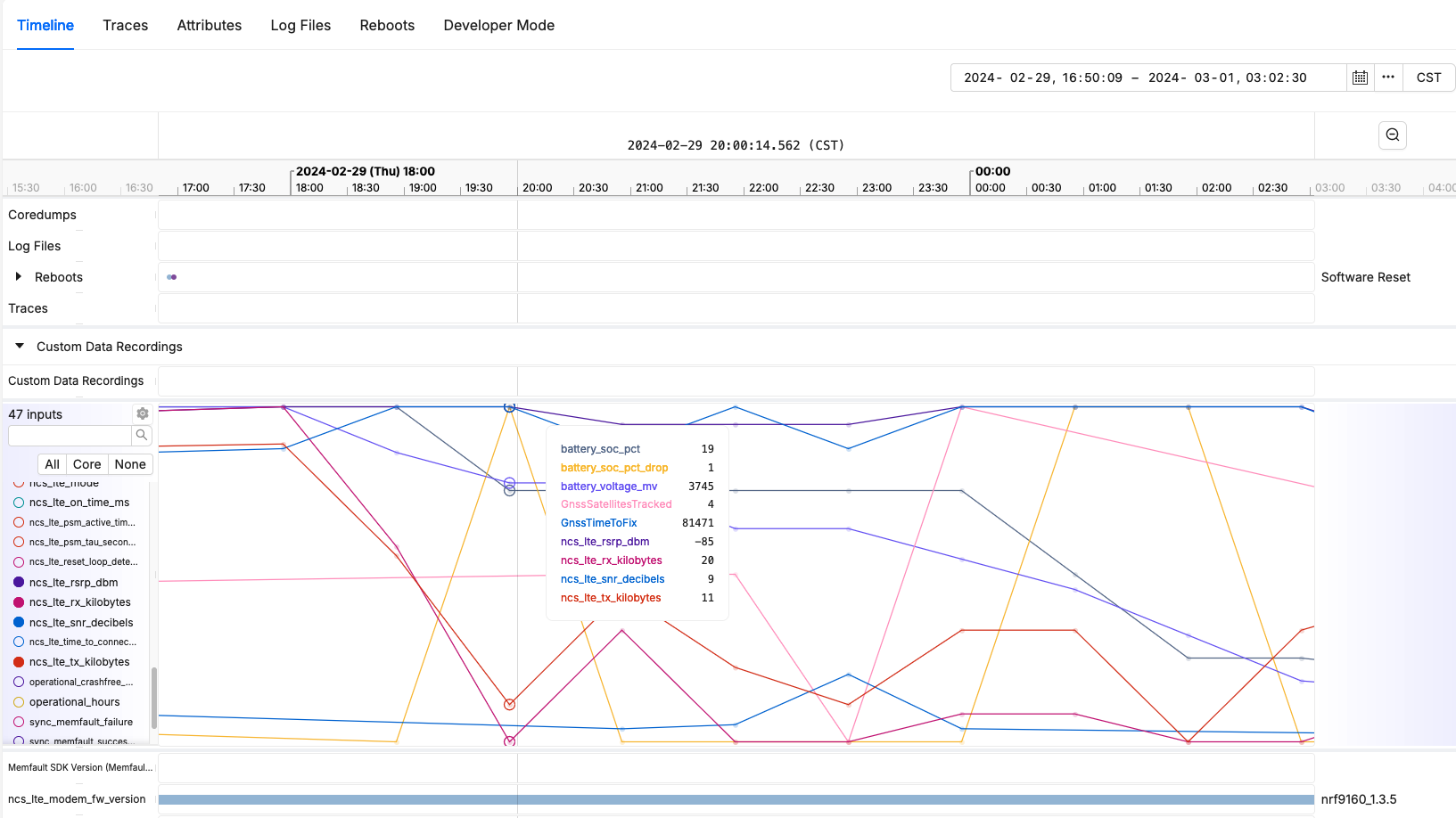
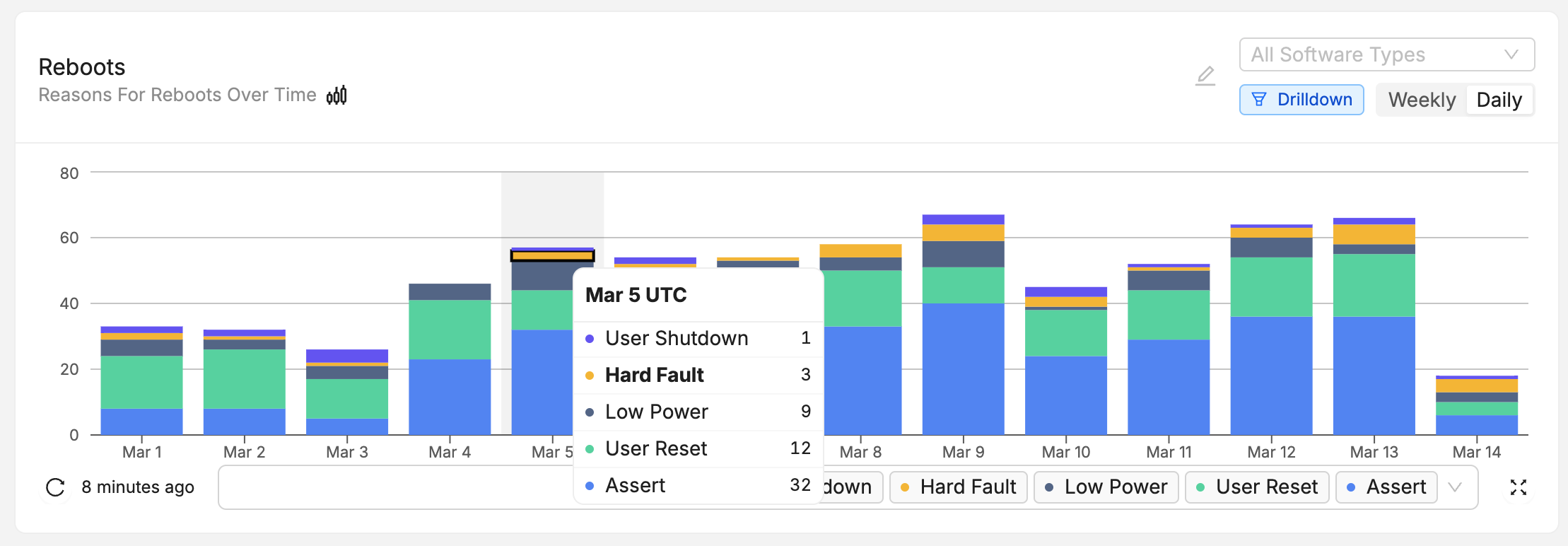
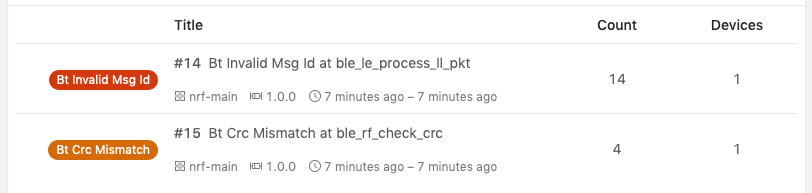
(Optionally) Collect a coredump capture using GDB
- Without GDB
- Using GDB
If you do not use GDB, skip ahead to the next step.
If you use GDB, a full coredump can be captured by just using the debugger! This can be useful during development to take advantage of Memfault's analyzers when a device crashes or hangs.
To perform the capture, navigate to http://app.memfault.com/ and select the "Issues" page in the Memfault UI, click on the "Manual Upload" button, and click on "walk-through on how to upload your first coredump". From there you can follow the guided steps to perform a capture. At the end you will see an analysis of your system state.
1. Create a Project
Create a Project and get a Project Key
Go to app.memfault.com and from the "Select A Project" dropdown, click on "Create Project" to setup your first project. Choose a name that reflects your product, such as "smart-sink-dev".
Once you've created your project, you'll be automatically taken to an page that includes your project key. Copy the key and follow the rest of this guide.
2. Set up the SDK
Prepare folder for memfault-firmware-sdk & port
The memfault-firmware-sdk
is a self-contained C SDK you will need to include
into your project.
Create a folder to hold memfault-firmware-sdk
as well as the configuration and
porting files to your platform.
cd ${YOUR_PROJECT_DIR}
mkdir -p third_party/memfault
cd third_party/memfault
# Add memfault repo as submodule, subtree, or copy in as source directly
git submodule add https://github.com/memfault/memfault-firmware-sdk.git memfault-firmware-sdk
cp memfault-firmware-sdk/ports/templates/* .
When you are done, you should have the following directory structure in your project:
memfault/
//...
├── memfault-firmware-sdk (submodule)
| # Files where port / glue layer to your platform will be implemented